In this tutorial, you will learn to create a simple Cookie Consent Popup in javaScript, HTML & CSS step by step. But before getting started, you should have a basic understanding of javascript cookies. So that you can easily add it to your website.
Cookies stores different types of user information as a text file in the web browser. So that, if users come back to the website, they will easily get the relevant information.
Therefore, Nowadays many websites use cookies for providing a better user experience. Even you have also seen the cookie consent alert box on various websites that ask to accept their cookies policy.
If You also need to use cookies on your website. then you will have to integrate a cookie consent notice. So that whenever users come to your website, they will get a cookie consent popup and accept the cookies policy.Because users must know about the cookie privacy & policy of your website.
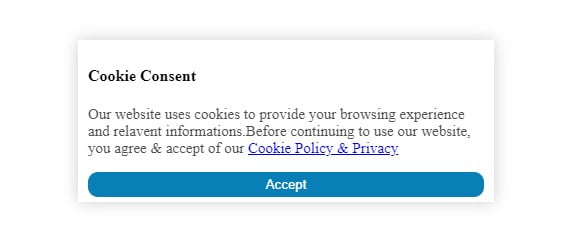
Steps to Create Cookie Consent Using Javascript
Before getting started coding, you should now understand the working of the cookie consent popup. So, let’s start
Learn also –
How to create JavaScript accordion
Export HTML Table Data to Excel File using JavaScript
Once you click the accept button to agree on cookies uses & cookies policy after the Cookie consent popup will not display again until the user remove the cookie of your website from the web browser or It is not expired.
If users block your website from the cookies settings in their web browser, your website can not be able to set cookies. then an alert notice will be appeared to allow all cookies site.
Here, I have set cookies for 30 days only. If you need to increase its expiration time, you can set it according to your website needs. Also, you can add your own CSS to the style cookie consent box.
1. Create Cookie Consent using HtML
Here, I have created a static cookie consent popup using HTML, Just copy it and paste it within the <body></body> element.
- Created a parent div with id=”cookiePopup”
- Also, created cookie content with heading or paragraph whatever you want to inform to users about cookie policy
- Then, Create a button to accept the cookie uses & policy by clicking it
<div id="cookiePopup"> <h4>Cookie Consent</h4> <p>Our website uses cookies to provide your browsing experience and relavent informations.Before continuing to use our website, you agree & accept of our <a href="#">Cookie Policy & Privacy</a></p> <button id="acceptCookie">Accept</button> </div>
2. Design Cookie Consent using CSS
Also, you have to use this CSS code in your website as internal or external without <style></style> element. You can also write your own css according to your website layout.
<style> #cookiePopup { background: white; width: 25%; position: fixed; left: 10px; bottom: 20px; box-shadow: 0px 0px 15px #cccccc; padding: 5px 10px; } #cookiePopup p{ text-align: left; font-size: 15px; color: #4e4e4e; } #cookiePopup button{ width: 100%; border: navajowhite; background: #097fb7; padding: 5px; border-radius: 10px; color: white; } </style>
3. Set Cookie to Browsers using JavaScript
Now, just copy this code and paste the above </body> tag or external file of your website then configure the following basic info according to you –
- cookieName=”Write here cookie name”
- cookieValue=” write here cookie value or content
- cookieExpireDays= “Write here no of days until cookie remains in browser “
Now, let’s understand the usage of created custom function –
createCookie – This function contains the code to set the cookie in the web browser and it will execute when users click the accept button
getCookie – This function contains the code to get the existing cookie from the web browser.
checkCookie – This function contains the code to check the created cookie using getcookie function. If cookie does not set then, the cookie consent will appear otherwise It will remain invisible.
<script type="text/javascript"> // set cookie according to you var cookieName= "CodingStatus"; var cookieValue="Coding Tutorials"; var cookieExpireDays= 30; // when users click accept button let acceptCookie= document.getElementById("acceptCookie"); acceptCookie.onclick= function(){ createCookie(cookieName, cookieValue, cookieExpireDays); } // function to set cookie in web browser let createCookie= function(cookieName, cookieValue, cookieExpireDays){ let currentDate = new Date(); currentDate.setTime(currentDate.getTime() + (cookieExpireDays*24*60*60*1000)); let expires = "expires=" + currentDate.toGMTString(); document.cookie = cookieName + "=" + cookieValue + ";" + expires + ";path=/"; if(document.cookie){ document.getElementById("cookiePopup").style.display = "none"; }else{ alert("Unable to set cookie. Please allow all cookies site from cookie setting of your browser"); } } // get cookie from the web browser let getCookie= function(cookieName){ let name = cookieName + "="; let decodedCookie = decodeURIComponent(document.cookie); let ca = decodedCookie.split(';'); for(let i = 0; i < ca.length; i++) { let c = ca[i]; while (c.charAt(0) == ' ') { c = c.substring(1); } if (c.indexOf(name) == 0) { return c.substring(name.length, c.length); } } return ""; } // check cookie is set or not let checkCookie= function(){ let check=getCookie(cookieName); if(check==""){ document.getElementById("cookiePopup").style.display = "block"; }else{ document.getElementById("cookiePopup").style.display = "none"; } } checkCookie(); </script>
4. See Cookie Consent Popup on Browser
After adding code successfully, When you open your website in the web browser, you will see a cookie consent popup. Once you click the accept button, It will not appear again until It will expire after 30 days or specified days by you.
Note – Cookie consent will not work if you open the page from anywhere in your local system. It will work only if placed in xampp/wamp server, live sever. You can also check it through the ngrock
5. See Saved Cookie in the Browser
When you click accept button, then you can see the saved cookie on the web browser as given in the following images
Step 1- Click the icon that remains at the right side of refresh icon
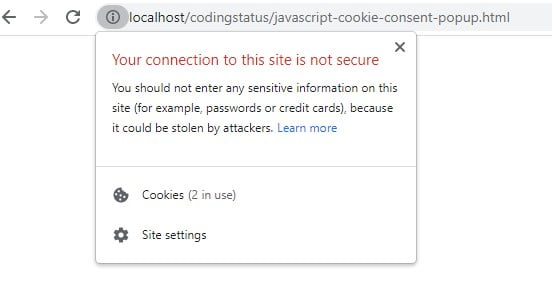
Step 2 – Click Cookies>localhost>CodingStatus
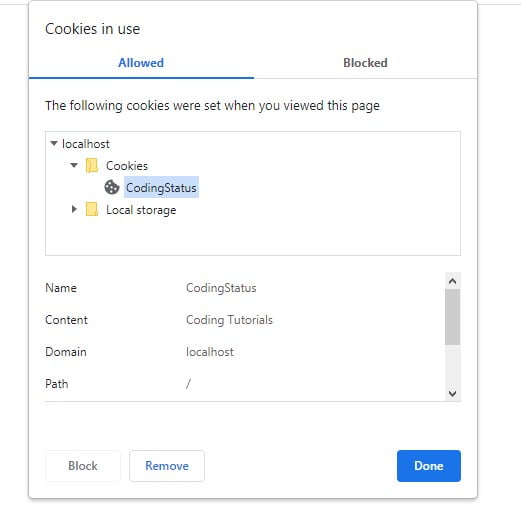